7 Python Tricks You Should Know
Impress your friends with these useful tips and tricks
There’s a treasure trove of useful Python tips and tricks online. Here are some fun, cool tricks you can use to beef up your Python game and impress your friends at the same time — kill two birds with one stone.
Without further ado, let’s jump right into it.
1. Download YouTube Videos With YouTube-Dl
You can easily download YouTube videos (and videos from many other websites) using the youtube-dl
module in Python.
First, let’s install the module using pip:
pip install youtube-dl
Once installed, you can download videos directly from terminal or command prompt by using the following one-line command:
youtube-dl <Your video link here>
Alternatively, since youtube-dl
has bindings for Python, you can create a Python script to do the same thing programmatically.
You can create a list with all the links and download the videos using the quick-and-dirty script below.
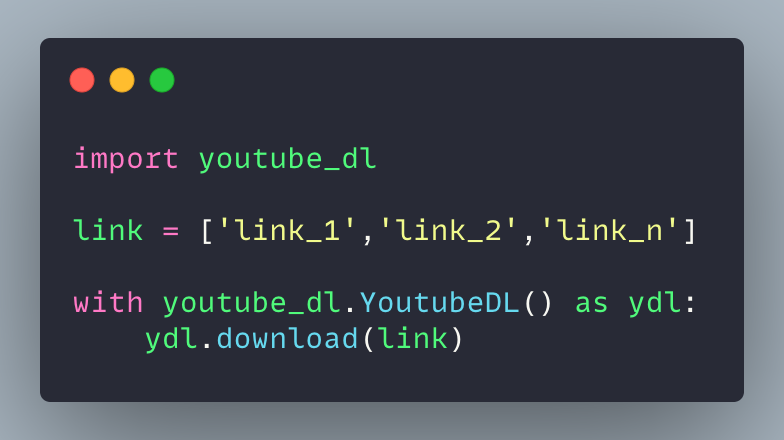
With this module, you can not only easily download videos, but entire playlists, metadata, thumbnails, subtitles, annotations, descriptions, audio, and much more.
The easiest way to achieve this is by adding a bunch of these parameters to a dictionary and passing it to the YoutubeDL
object constructor.
In the code below I created a dictionary, ydl_options
, with some parameters, and passed it on to the constructor:
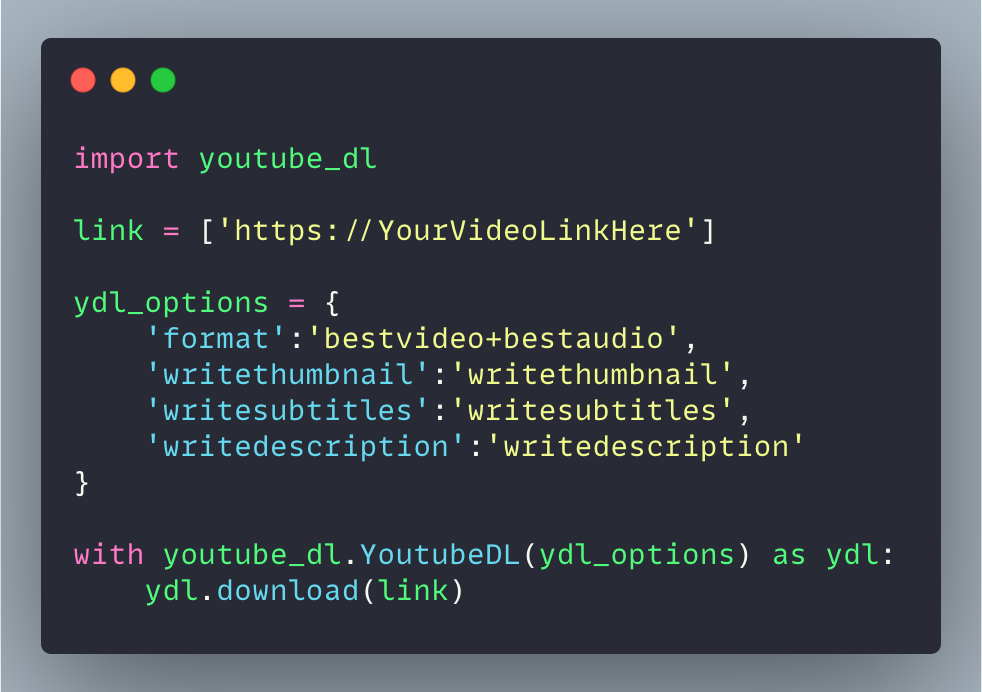
1. 'format':'bestvideo+bestaudio' #Dowloads the video in the best available video and audio format.2. 'writethumbnail':'writethumbnail' #Downloads the thumbnail image of the video.3. 'writesubtitles':'writesubtitles' #Downloads the subtitles, if any.4. 'writedescription':'writedescription' #Writes the video description to a .description file.
Note: You can do everything directly within the terminal or a command prompt, but using a Python script is better due to the flexibility/reusability it offers.
You can find more details about the module here: Github:youtube-dl.
2. Debug Your Code With Pdb
Python has its own in-built debugger called pdb. A debugger is an extremely useful tool that helps programmers to inspect variables and program execution, line by line. A debugger means programmers don’t have to pull their hair out trying to find pesky issues in their code.
The good thing about pdb is that it is included with the standard Python library. As a result, this beauty can be used on any machine where Python is installed. This comes in handy in environments with restrictions on installing any add-on on top of the vanilla Python installation.
There are several ways to invoke the pdb debugger:
In-line breakpoint
pdb.set_trace()In Python 3.7 and later
breakpoint()pdb.py can also be invoked as a script to debug other scripts
python3 -m pdb myscript.py
Here’s a sample code on Python 3.8 that invokes pdb using the breakpoint()
function:
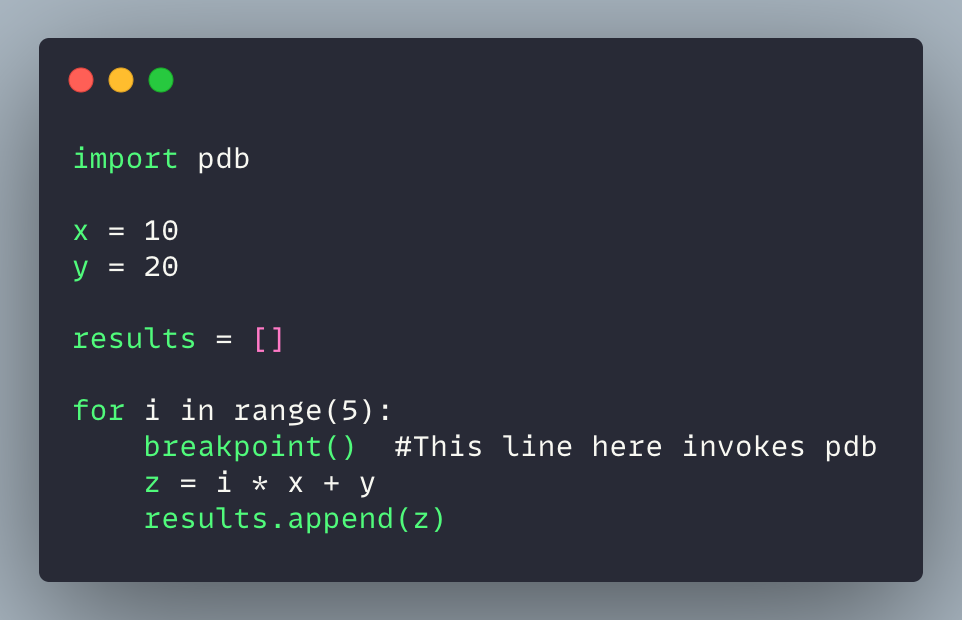
Here are some of the most useful commands to aid you in your debugging adventure:
n
: To continue execution until the next line in the current function is reached or it returns.l
: list codej <line>
: jump to a lineb <line>
: set breakpoint()c
: continue until breakpointq
: quit
Note: Once you are in pdb, n
, l
, b
, c
, and q
become reserved keywords. The last one will quit pdb if you have a variable named q
in your code.
You can find more details about this here: pdb — The Python Debugger
3. Make Your Python Code Into an Executable File Using PyInstaller
Not a lot of people know this, but you can convert your Python scripts into standalone executables. The biggest benefit to this is that your Python scripts/applications can then work on machines where Python (and any necessary third-party packages) are not installed.
PyInstaller works on pretty much all the major platforms, including Windows, GNU/Linux, Mac OS X, FreeBSD, Solaris and AIX.
To install it, use the following command in pip:
pip install pyinstaller
Then, go to the directory where your program is located and run:
pyinstaller myscript.py
This will generate the executable and place it in a subdirectory called dist
.
PyInstaller provides many options for customization:
pyinstaller --onefile --icon [icon file] [script file]# Using the --onefile option bundles everything in a single executable file instead of having a bunch of other files.
# Using the --icon option adds a custom icon (.ico file) for the executable file
Pyinstaller is compatible with most of the third-party packages — Django, NumPy, Matplotlib, SQLAlchemy, Pandas, Selenium, and many more.
To learn about all the features and the myriad of options Pyinstaller provides, visit its page on Github: Pyinstaller.
4. Make a Progress Bar With Tqdm
The TQDM library will let you create fast, extensible progress bars for Python and CLI.
You’d need to first install the module using pip:
pip install tqdm
With a few lines of code, you can add smart progress bars to your Python scripts.
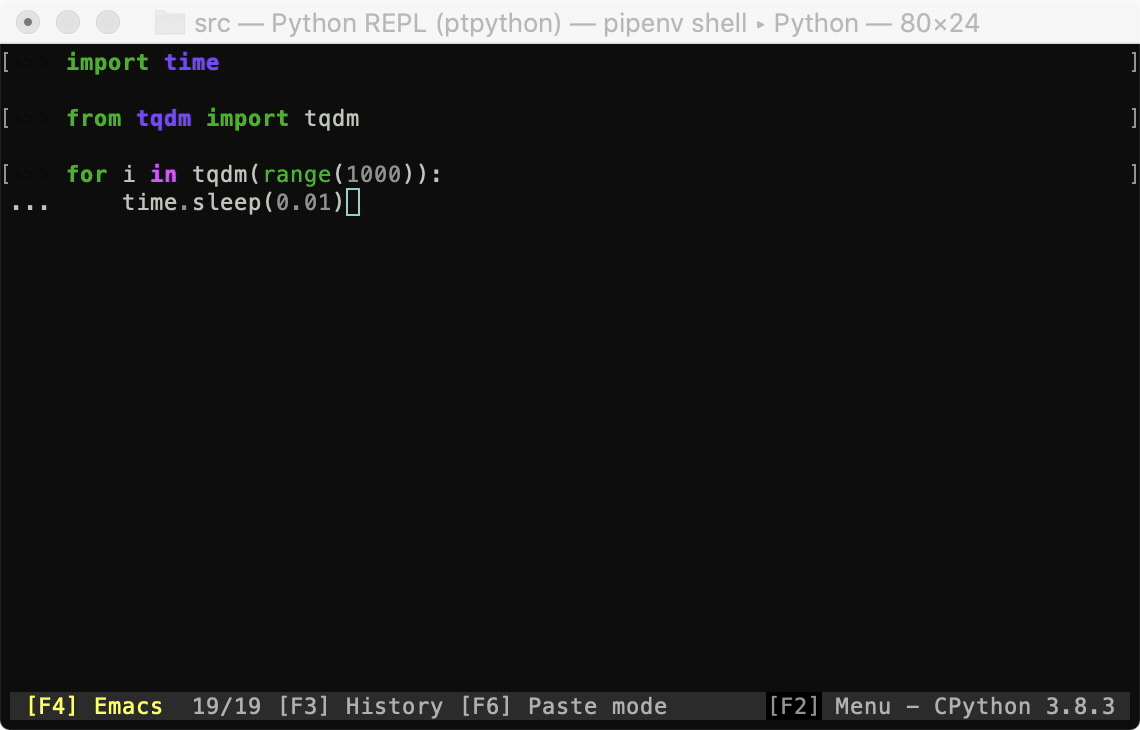
TQDM works on all major platforms like Linux, Windows, Mac, FreeBSD, NetBSD, Solaris/SunOS. Not only that, but it also integrates seamlessly with any console, GUI, and IPython/Jupyter notebooks.
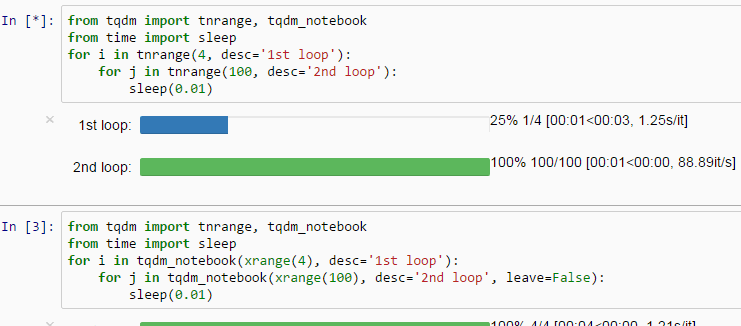
To get more details on all the tricks tqdm has up its sleeve, visit its official page here: tqdm.
5. Add Color to Your Console Output With Colorama
Colorama is a nifty little cross-platform module that adds color to the console output. Let’s install it using pip:
pip install colorama
Colorama provides the following formatting constants:
Fore: BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET.
Back: BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET.
Style: DIM, NORMAL, BRIGHT, RESET_ALL
Here’s a sample code to use Colorama:
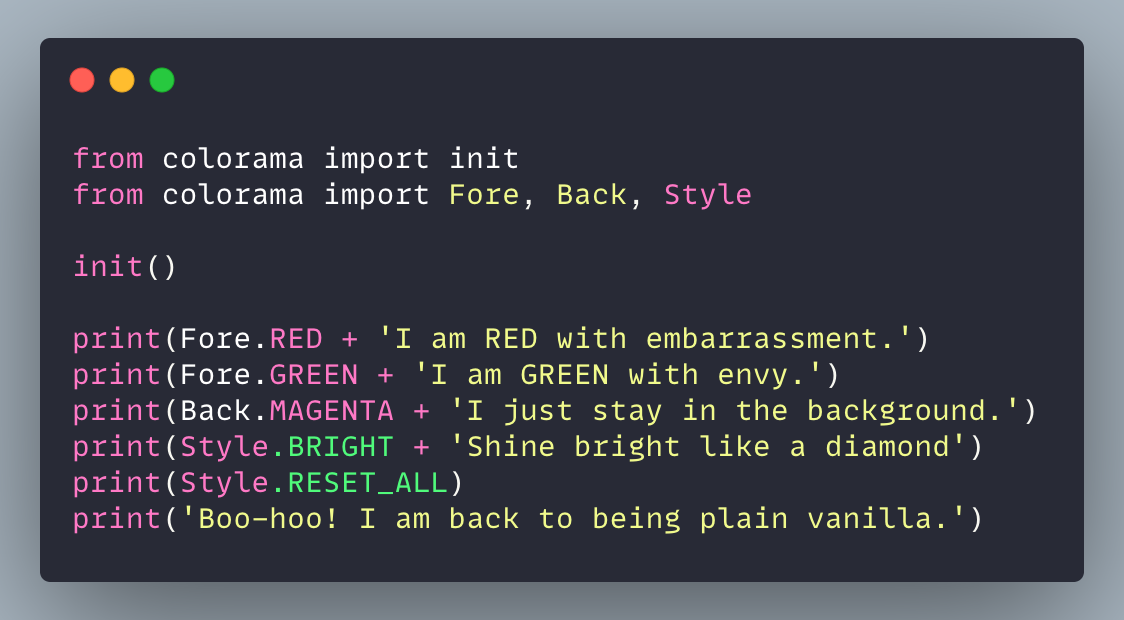
The code above yields the following output:
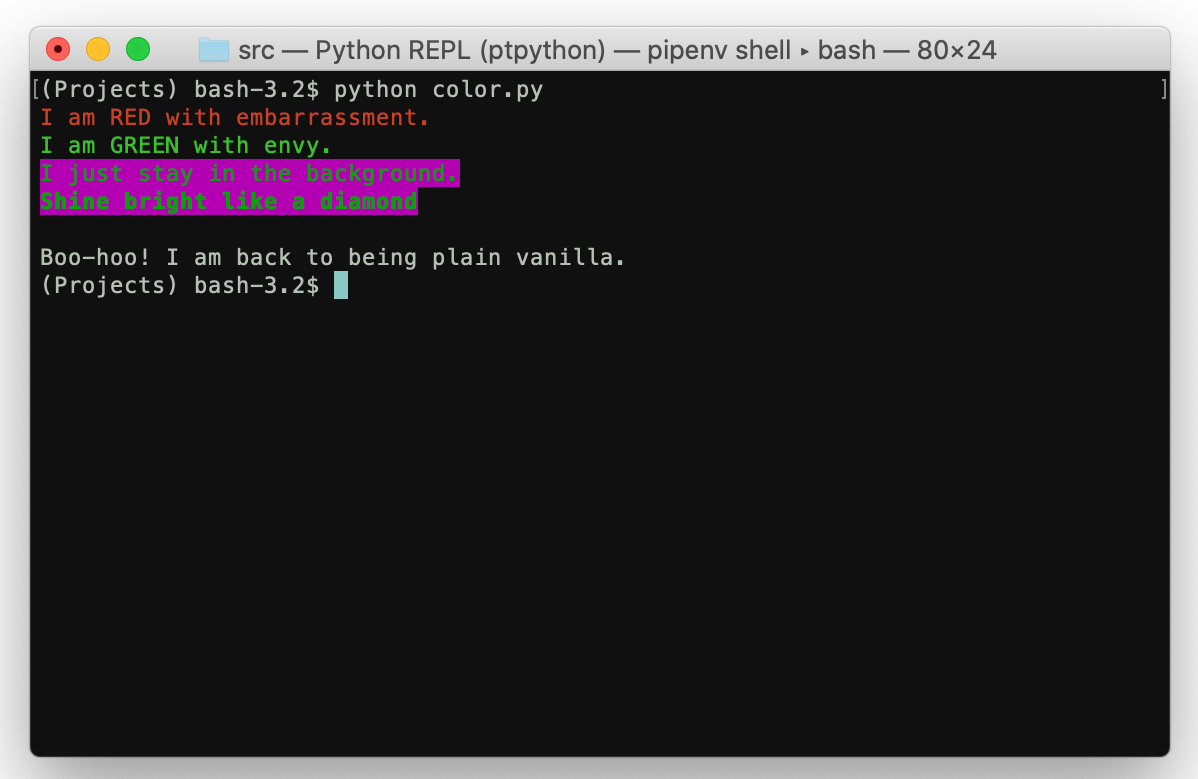
Style.RESET_ALL
explicitly resets the foreground, background, and brightness — although, Colorama also performs this reset automatically on program exit.
Colorama has other features that you can find here: Colorama Website.
6. Pretty Print 2D Lists Using Tabulate
Often, dealing with tabular output in Python is a pain. That’s when tabulate comes to the rescue. It can transform your output from “The output looks like hieroglyphs to me?” to “Wow, that looks pretty!”. Well, maybe that last part is a slight exaggeration, but it will improve the readability of your output.
First, install it using pip:
pip install tabulate
Here’s a simple snippet to print a 2D list as a table using tabulate:
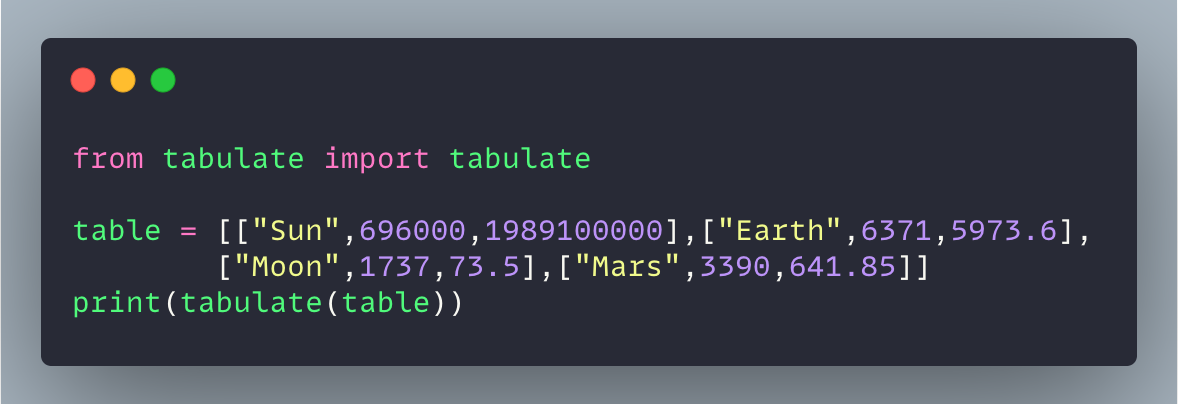
The GIF below shows how the output of the code above looks with and without tabulate. No prizes for guessing which of the two outputs is more readable!
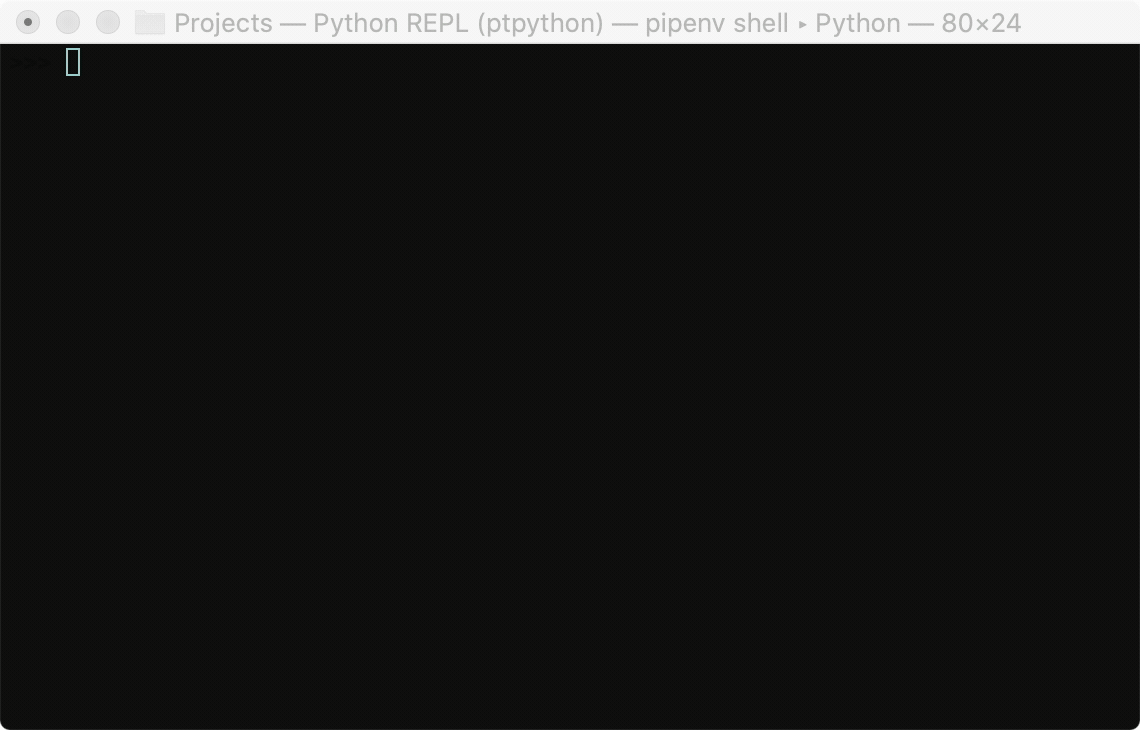
Tabulate supports the following data types:
1. list of lists or another iterable of iterables
2. list or another iterable of dicts (keys as columns)
3. dict of iterables (keys as columns)
4. two-dimensional NumPy array
5. NumPy record arrays (names as columns)
6. pandas.DataFrameSource: https://pypi.org/project/tabulate/
Here’s an example that works on a dictionary:
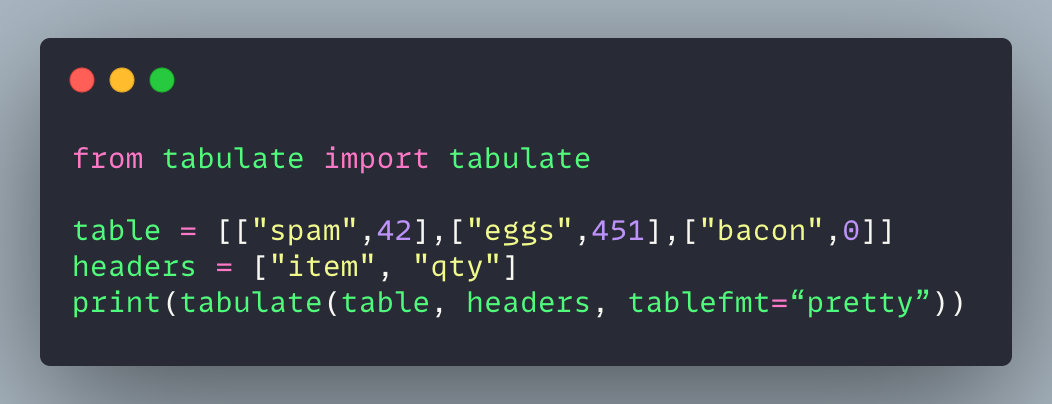
This pretty-prints the dictionary:
+-------+-----+
| item | qty |
+-------+-----+
| spam | 42 |
| eggs | 451 |
| bacon | 0 |
+-------+-----+
You can find more details about the library here: tabulate.
7. Spruce Up Your Standard Python Shell With Ptpython
In case you’re wondering why my Python shell is sexier than yours, it’s because I’ve been using a custom Python shell. This shell, ptpython, has a lot of enhancements over the standard Python shell. Basically, if the standard Python shell and ptpython were twins, the latter would be the prettier (and more successful) of the two siblings.
You can install ptpython through pip:
pip install ptpython
Once installed, you can invoke it by typing ptpython
in your standard shell.
It has several features over the standard shell:
1. Code indentation
2. Syntax highlighting
3. Autocompletion
4. Multiline editing
5. Support for color schemes
... and many other things
In the GIF below, you can see features 1–3 in action:
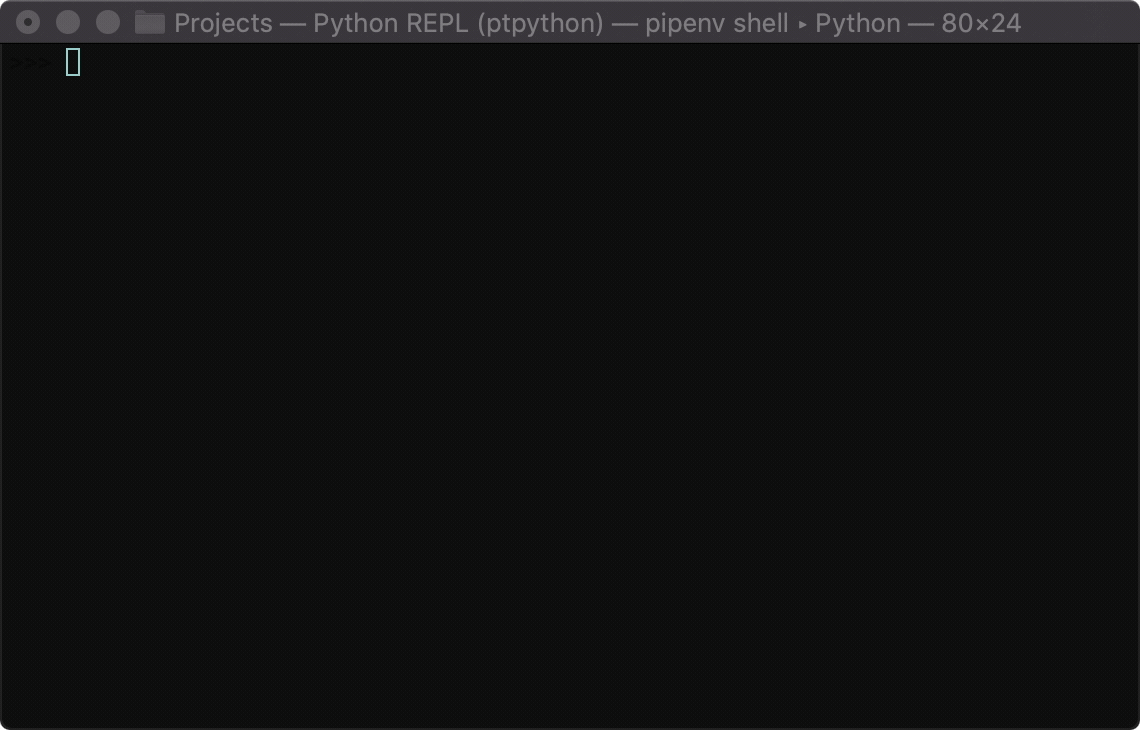
To learn more about its features, visit its website here: ptpython.
I hope you enjoyed the article and learned something new in the process.
Do you have any cool Python tricks? Chime in with yours in the comments.
'Data Analytics(en)' 카테고리의 다른 글
Advanced Python: 9 Best Practices to Apply When You Define Classes (0) | 2020.10.24 |
---|---|
Tutorial: Stop Running Jupyter Notebooks from your Command Line! (0) | 2020.10.23 |
Advanced Python: Consider These 10 Elements When You Define Python Functions (0) | 2020.10.21 |
ROCKET: Fast and Accurate Time Series Classification (0) | 2020.10.20 |
The Beginner’s Guide to Pydantic (0) | 2020.10.19 |